!date
mardi 29 janvier 2019, 13:53:10 (UTC+0100)
Modifying the segment natureΒΆ
This section illustrates a simple ray tracing simulation. The environement is a building with 2 rooms. At the interface between the two rooms there is a segment with 3 sub-segment whose material properties are changed in the simulation. This section illustrates in details the different steps of the simulation. First we need to import several specialized modules.
from pylayers.simul.link import *
from pylayers.antprop.rays import *
from pylayers.antprop.aarray import *
from pylayers.antprop.channel import *
from pylayers.gis.layout import *
from pylayers.antprop.signature import *
import pylayers.signal.bsignal as bs
import pylayers.signal.waveform as wvf
import matplotlib.pyplot as plt
%matplotlib inline
Lets start by loading a simple layout with 2 single rooms. The multi subsegment appears in the middle with the red vertical lines. Each subsegment is materialized by a segment.
L=Layout('defstr.lay')
f,a=L.showG('s',subseg=True,figsize=(10,10))
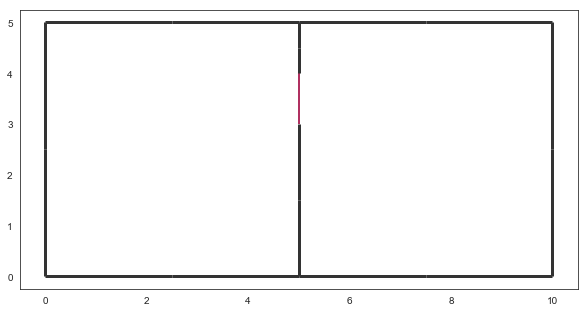
The attribute lsss
lists all the segments which are superposed
called iso
segment.
L.lsss
[1, 2, 3]
The studied configuration is composed of a simple 2 rooms building separated by an iso segment.
print(L.Gs.node[1])
print(L.Gs.node[2])
print(L.Gs.node[3])
{'transition': False, 'iso': [3, 2], 'ncycles': [], 'connect': [-8, -7], 'norm': array([ 1., -0., 0.]), 'z': (0.0, 2.7), 'offset': 0, 'name': 'WOOD'}
{'transition': False, 'iso': [1, 3], 'ncycles': [], 'connect': [-8, -7], 'norm': array([ 1., -0., 0.]), 'z': (2.7, 2.8), 'offset': 0, 'name': 'AIR'}
{'transition': False, 'iso': [1, 2], 'ncycles': [], 'connect': [-8, -7], 'norm': array([ 1., -0., 0.]), 'z': (2.8, 3), 'offset': 0, 'name': 'WOOD'}
The \(\mathcal{G}_s\) graph dictionary has the following node. Negative nodes are points and positive index nodes are segments.
for k in [-1,1,2]:
print(L.Gs.node[k])
{}
{'transition': False, 'iso': [3, 2], 'ncycles': [], 'connect': [-8, -7], 'norm': array([ 1., -0., 0.]), 'z': (0.0, 2.7), 'offset': 0, 'name': 'WOOD'}
{'transition': False, 'iso': [1, 3], 'ncycles': [], 'connect': [-8, -7], 'norm': array([ 1., -0., 0.]), 'z': (2.7, 2.8), 'offset': 0, 'name': 'AIR'}
We define now, the position of the transmitter and the receiver, the two termination of a radio link.
#tx=np.array([759,1114,1.5])
#rx=np.array([767,1114,1.5])
tx=np.array([2,3,1.5])
rx=np.array([8,4,1.6])
fGHz=np.linspace(1,11,100)
#Aa = Antenna('S1R1.vsh3')
#Ab = Antenna('S1R1.vsh3')
Aa = Antenna('Gauss',fGHz=fGHz)
Ab = Antenna('Gauss',fGHz=fGHz)
#Aa = AntArray(N=[8,1,1],fGHz=fGHz)
#Ab = AntArray(N=[4,1,1],fGHz=fGHz)
Lk = DLink(L=L,
a=tx,
b=rx,
Aa=Aa,
Ab=Ab,
fGHz=fGHz,
cutoff=5)
Lk.eval(force=True,verbose=False)
Signatures: 100.00000000000001it [00:00, 1634.19it/s]
Warning Unable to read graph Gr
Warning Unable to read graph Gw
Layout Graph loaded
Aa.eval()
Aa.plotG()
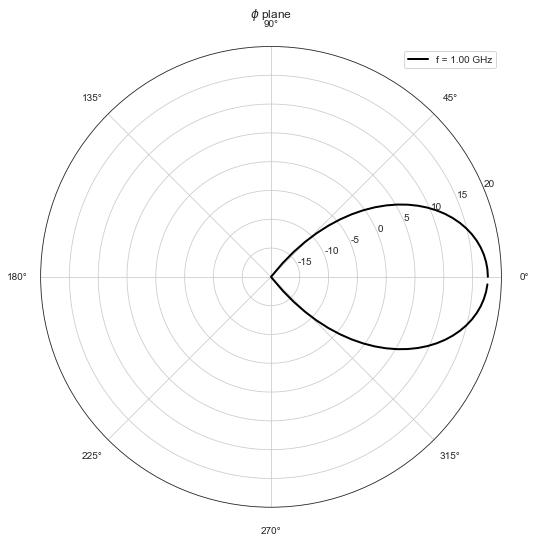
(<matplotlib.figure.Figure at 0x7fa361a69dd8>,
<matplotlib.projections.polar.PolarAxes at 0x7fa3dd381fd0>)
Lk.C.Ctt
FUsignal : (100,) (25, 100)
Lk.eval(force=True,a=tx,b=rx,applywav=True)
Signatures: 100.00000000000001it [00:00, 1986.58it/s]
/home/uguen/Documents/rch/devel/pylayers/pylayers/signal/bsignal.py:1087: VisibleDeprecationWarning: converting an array with ndim > 0 to an index will result in an error in the future
pstop2 = pstart2 + np.where(u2.x[pstart2:dim2] == xnew[-1])[0]
/home/uguen/Documents/rch/devel/pylayers/pylayers/signal/bsignal.py:1202: VisibleDeprecationWarning: converting an array with ndim > 0 to an index will result in an error in the future
x_new = self.x[imin:imax]
/home/uguen/Documents/rch/devel/pylayers/pylayers/signal/bsignal.py:1207: VisibleDeprecationWarning: converting an array with ndim > 0 to an index will result in an error in the future
y_new = self.y[..., imin:imax]
/home/uguen/Documents/rch/devel/pylayers/pylayers/antprop/channel.py:4627: VisibleDeprecationWarning: using a non-integer number instead of an integer will result in an error in the future
rir = np.zeros((shy[0],N))
Lk.C
Ctilde : Ray Propagation Channel Tensor (2x2xrxf)
---------
between antennas local frames
Nray : 25
Nfreq : 100
fmin(GHz) : 1.0
fmax(GHz): 11.0
---1st ray 1st freq ---
global angles (th,ph) degrees :
[ 89.1 9.5]
[ 90.9 -170.5]
local angles (th,ph) degrees :
[ 89.1 9.5]
[ 90.9 -170.5]
| (0.110598827081-0.0932603392219j) (7.67365332983e-05-9.12653903417e-05j) |
| (7.67365332983e-05-9.12653903417e-05j) (0.10982834-0.0923439725161j) |
f = plt.figure(figsize=(10,10))
f,a=Lk.C.show(cmap='jet',fig=f,typ='l20',vmin=-120,vmax=-10)
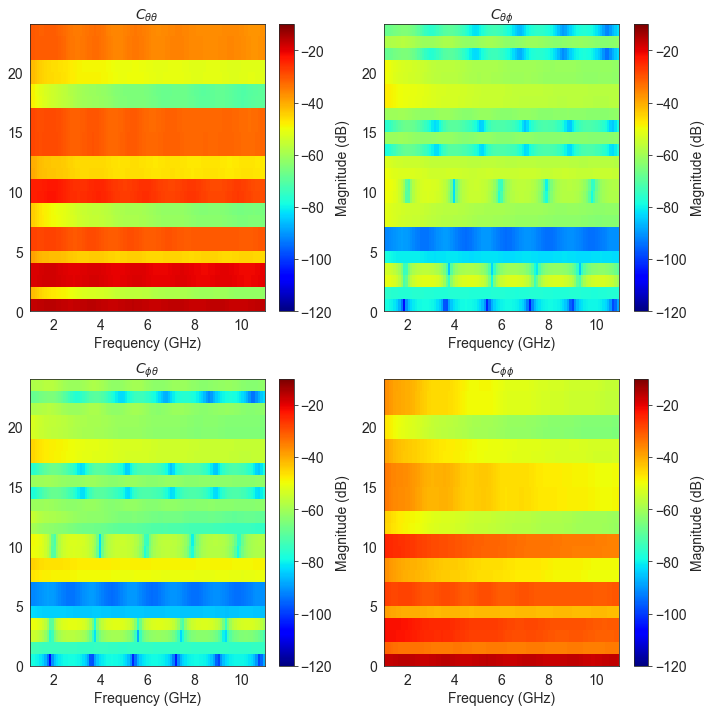
fGHz=np.arange(2,6,0.1)
wav = wvf.Waveform(fcGHz=4,bandGHz=1.5)
wav.show()
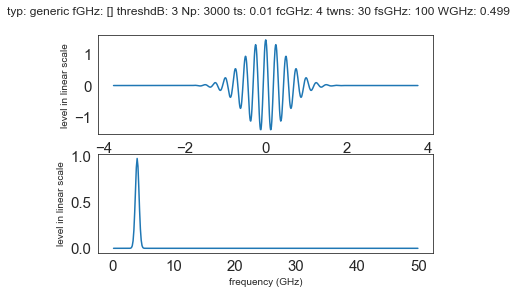
Lk = DLink(L=L,a=tx,b=rx,fGHz=fGHz)
Warning Unable to read graph Gr
Warning Unable to read graph Gw
Layout Graph loaded
Position of the transmitter
Lk.a
array([ 2. , 3. , 1.5])
Position of the receiver
Lk.b
array([ 8. , 4. , 1.6])
cir = Lk.H.applywavB(wav.sf)
WARNING : Tchannel.applywavB is going to be replaced by Tchannel.applywav
/home/uguen/Documents/rch/devel/pylayers/pylayers/signal/bsignal.py:1074: VisibleDeprecationWarning: converting an array with ndim > 0 to an index will result in an error in the future
pstop1 = pstart1 + np.where(u1.x[pstart1:dim1]==xnew[-1])[0]
/home/uguen/Documents/rch/devel/pylayers/pylayers/signal/bsignal.py:1202: VisibleDeprecationWarning: converting an array with ndim > 0 to an index will result in an error in the future
x_new = self.x[imin:imax]
/home/uguen/Documents/rch/devel/pylayers/pylayers/signal/bsignal.py:1207: VisibleDeprecationWarning: converting an array with ndim > 0 to an index will result in an error in the future
y_new = self.y[..., imin:imax]
L.Gs.node[1]['name']='AIR'
L.Gs.node[2]['name']='AIR'
L.Gs.node[3]['name']='AIR'
#Aa = Antenna('Omni',fGHz=fGHz)
#Ab = Antenna('Omni',fGHz=fGHz)
Lk.eval(force=True,verbose=0,fGHz=fGHz)
Lk.plt_cir()
plt.xlim(0,50)
plt.title('AIR/AIR/AIR')
plt.figure()
L.Gs.node[1]['name']='METAL'
L.Gs.node[2]['name']='METAL'
L.Gs.node[3]['name']='METAL'
Lk.eval(force=True,verbose=0,fGHz=fGHz)
Lk.plt_cir()
plt.xlim(0,50)
plt.title('METAL/METAL/METAL')
plt.figure()
L.Gs.node[1]['name']='WOOD'
L.Gs.node[2]['name']='AIR'
L.Gs.node[3]['name']='WOOD'
Lk.eval(force=True,verbose=0,fGHz=fGHz)
Lk.plt_cir()
plt.xlim(0,50)
plt.title('WOOD/AIR/WOOD')
Signatures: 100.00000000000001it [00:00, 968.00it/s]
Signatures: 100.00000000000001it [00:00, 2984.53it/s]
/home/uguen/Documents/rch/devel/pylayers/pylayers/simul/link.py:2489: RuntimeWarning: divide by zero encountered in log10
ERdB = 10*np.log10(ER)
Signatures: 100.00000000000001it [00:00, 2006.53it/s]
<matplotlib.text.Text at 0x7fa35b746588>
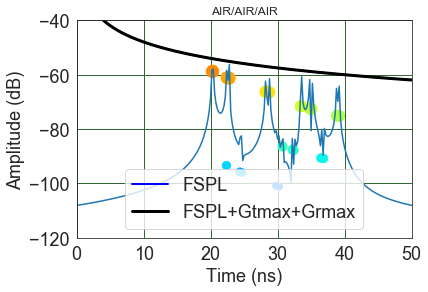
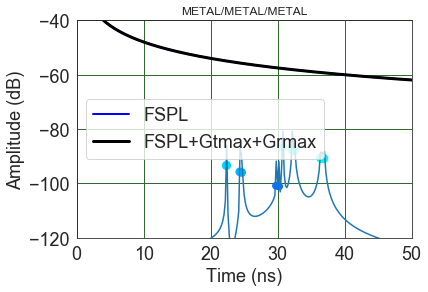
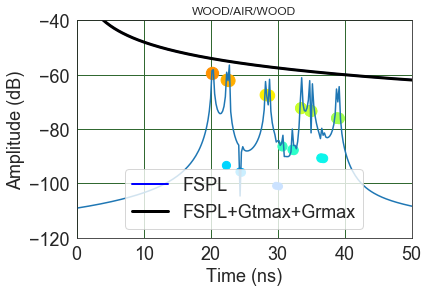
We have modified successively the nature of the 3 surfaces in the sub
segment placed in the separation partition. The first was AIR, the
second WOOD and the third METAL. As the subsegment is placed on the LOS
path the blockage effect is clearly visible. The chosen antennas were
omni directional Antenna('Omni')